1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
|
# Epub.js v0.3
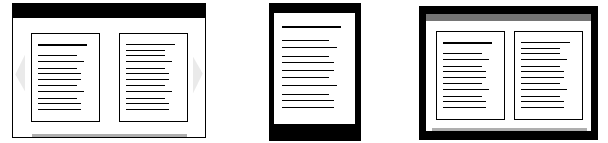
Epub.js is a JavaScript library for rendering ePub documents in the browser, across many devices.
Epub.js provides an interface for common ebook functions (such as rendering, persistence and pagination) without the need to develop a dedicated application or plugin. Importantly, it has an incredibly permissive [Free BSD](http://en.wikipedia.org/wiki/BSD_licenses) license.
[Try it while reading Moby Dick](https://futurepress.github.io/epubjs-reader/)
## Why EPUB

The [EPUB standard](http://www.idpf.org/epub/30/spec/epub30-overview.html) is a widely used and easily convertible format. Many books are currently in this format, and it is convertible to many other formats (such as PDF, Mobi and iBooks).
An unzipped EPUB3 is a collection of HTML5 files, CSS, images and other media – just like any other website. However, it enforces a schema of book components, which allows us to render a book and its parts based on a controlled vocabulary.
More specifically, the EPUB schema standardizes the table of contents, provides a manifest that enables the caching of the entire book, and separates the storage of the content from how it’s displayed.
## Getting Started
Get the minified code from the build folder:
```html
<script src="../dist/epub.min.js"></script>
```
If using archived `.epub` files include JSZip:
```html
<script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.1.5/jszip.min.js"></script>
```
Set up a element to render to:
```html
<div id="area"></div>
```
Create the new ePub, and then render it to that element:
```html
<script>
var book = ePub("url/to/book/package.opf");
var rendition = book.renderTo("area", {width: 600, height: 400});
var displayed = rendition.display();
</script>
```
## Render Methods
### Default
```js
book.renderTo("area", { method: "default", width: "100%", height: "100%" });
```
[View example](http://futurepress.github.io/epub.js/examples/spreads.html)
The default manager only displays a single section at a time.
### Continuous
```js
book.renderTo("area", { method: "continuous", width: "100%", height: "100%" });
```
[View example](http://futurepress.github.io/epub.js/examples/continuous-scrolled.html)
The continuous manager will display as many sections as need to fill the screen, and preload the next section offscreen. This enables seamless swiping / scrolling between pages on mobile and desktop, but is less performant than the default method.
## Flow Overrides
### Auto (Default)
`book.renderTo("area", { flow: "auto", width: "900", height: "600" });`
Flow will be based on the settings in the OPF, defaults to `paginated`.
### Paginated
```js
book.renderTo("area", { flow: "paginated", width: "900", height: "600" });
```
[View example](http://futurepress.github.io/epub.js/examples/spreads.html)
Scrolled: `book.renderTo("area", { flow: "scrolled-doc" });`
[View example](http://futurepress.github.io/epub.js/examples/scrolled.html)
## Documentation
API documentation is available at [epubjs.org/documentation/0.3/](http://epubjs.org/documentation/0.3/)
A Markdown version is included in the repo at [documentation/API.md](https://github.com/futurepress/epub.js/blob/master/documentation/md/API.md)
## Running Locally
install [node.js](http://nodejs.org/)
Then install the project dependences with npm
```javascript
npm install
```
You can run the reader locally with the command
```javascript
npm start
```
## Examples
+ [Spreads](http://futurepress.github.io/epub.js/examples/spreads.html)
+ [Scrolled](http://futurepress.github.io/epub.js/examples/scrolled.html)
+ [Swipe](http://futurepress.github.io/epub.js/examples/swipe.html)
+ [Input](http://futurepress.github.io/epub.js/examples/input.html)
+ [Highlights](http://futurepress.github.io/epub.js/examples/highlights.html)
[View All Examples](http://futurepress.github.io/epub.js/examples/)
## Testing
Test can be run by Karma from NPM
```js
npm test
```
## Building for Distribution
Builds are concatenated and minified using [webpack](https://webpack.js.org/) and [babel](https://babeljs.io/)
To generate a new build run
```javascript
npm run prepare
```
or to continuously build run
```javascript
npm run watch
```
## Hooks
Similar to a plugins, Epub.js implements events that can be "hooked" into. Thus you can interact with and manipulate the contents of the book.
Examples of this functionality is loading videos from YouTube links before displaying a chapter's contents or implementing annotation.
Hooks require an event to register to and a can return a promise to block until they are finished.
Example hook:
```javascript
rendition.hooks.content.register(function(contents, view) {
var elements = contents.document.querySelectorAll('[video]');
var items = Array.prototype.slice.call(elements);
items.forEach(function(item){
// do something with the video item
});
})
```
The parts of the rendering process that can be hooked into are below.
```js
book.spine.hooks.serialize // Section is being converted to text
book.spine.hooks.content // Section has been loaded and parsed
rendition.hooks.render // Section is rendered to the screen
rendition.hooks.content // Section contents have been loaded
rendition.hooks.unloaded // Section contents are being unloaded
```
## Reader
The reader has moved to its own repo at: https://github.com/futurepress/epubjs-reader/
## Additional Resources
[](https://gitter.im/futurepress/epub.js "Gitter Chat")
[Epub.js Developer Mailing List](https://groups.google.com/forum/#!forum/epubjs)
IRC Server: freenode.net Channel: #epub.js
Follow us on twitter: @Epubjs
+ http://twitter.com/#!/Epubjs
## Other
EPUB is a registered trademark of the [IDPF](http://idpf.org/).
|